Input | UI components (BETA)
APPLICABLE PRODUCTS
Sales Hub
- Enterprise
Service Hub
- Enterprise
The Input
component renders a text input field where a user can enter a custom text value. Like other inputs, this component should only be used within a Form that has a submit button.
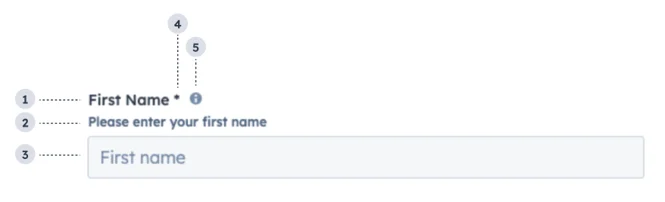
- Label: the input's label.
- Description: the text that describes the field's purpose.
- Placeholder: the placeholder value that displays when no value has been entered.
- Required field indicator: communicates to the user that the field is required for form submission.
- Tooltip: on hover, displays additional information about the field.
Prop | Type | Description |
---|---|---|
name Required |
String | The input's unique identifier, similar to the HTML input element name attribute. |
label Required |
String | The text that displays above the input. Required if inputType is not set to hidden . |
required |
Boolean | When set to true , displays a required field indicator. |
value |
Function | The value of the input. |
type |
'text' (default) | 'password' |
The type of input. An input with the 'password' type will hide the characters that the user types. |
readOnly |
Boolean | When set to true , users will not be able to enter a value into the field. |
description |
String | Displayed text that describes the field's purpose. |
tooltip |
String | The text that displays in a tooltip next to the input label. |
placeholder |
String | The text that appears in the input before a value is set. |
error |
Boolean | When set to true , validationMessage is displayed as an error message if provided. The input will also render in an error state so that users are aware. If left false , validationMessage is displayed as a success message. |
validationMessage |
String | The text to show if the input has an error. |
onChange |
(value: string) => void | A callback function that is invoked when the value is committed. Currently, these are onBlur of the input and when the user submits the form. |
onInput |
(value: string) => void | A function that is called and passes the value when the field is edited by the user. Should be used for validation. It's recommended that you don't use this value to update state (use onChange instead). |
onBlur |
(value: string) => void | A function that is called and passes the value when the field loses focus. |
onFocus |
(value: string) => void | A function that is called and passed the value when the field gets focused. |
Use for form fields where a user can enter any text value, such as email address or name.
- DO: make label and description text concise and clear.
- DO: include placeholder text to help users understand what's expected in the field.
- DO: indicate if a field is required.
- DO: include clear validation error messages so that users know how to fix errors.
- DON'T: use this component for long responses, such as open-ended comments or feedback. Instead, use the TextArea component.
- DON'T: use placeholder text for critical information, as it will disappear once users begin to type. Critical information should be placed in the label and descriptive text, with additional context in the tooltip if needed.
Thank you for your feedback, it means a lot to us.
This form is used for documentation feedback only. Learn how to get help with HubSpot.