NumberInput | UI components (BETA)
APPLICABLE PRODUCTS
Sales Hub
- Enterprise
Service Hub
- Enterprise
The NumberInput
component renders a number input field. Commonly used within the Form component.
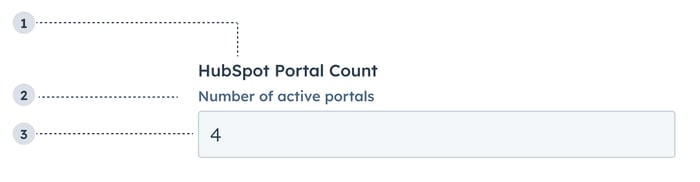
- Label: the input's label.
- Description: the text that describes the field's purpose.
- Value: an entered value.
Prop | Type | Description |
---|---|---|
name Required |
String | The input's unique identifier. |
label Required |
String | The text that displays above the dropdown menu. |
value |
String | number | The value of the input. |
defaultValue |
Number | The value of the input on initial render. |
description |
String | Text that describes the field's purpose. |
required |
Boolean | When set to true , displays a required field indicator. |
readOnly |
Boolean | When set to true , users will not be able to enter a value into the field. Set to false by default. |
placeholder |
String | The text that appears in the input before a value is set. |
tooltip |
String | The text that displays in a tooltip next to the label. |
error |
Boolean | When set to true , validationMessage is displayed as an error message if provided. The input will also render its error state to let the user know there's an error. When false (default), validationMessage is displayed as a success message. |
validationMessage |
String | The text to display if the input has an error. |
min |
Number | Sets the lower bound of the input. |
max |
Number | Sets the upper bound of the input. |
precision |
Number | Sets the number of digits to the right of the decimal point. |
formatStyle |
'decimal' | 'percentage' |
Formats the input as a decimal or percentage. |
onBlur |
(value: number) => void | A function that is called every time the field loses focus, passing the value. |
onChange |
(value: number) => void | A callback function that is invoked when the value is committed. Currently these times are onBlur of the input and when the user submits the form. |
onFocus |
(value: number) => void | A function that is called every time the field gets focused on, passing the value. |
A field in a form where salespeople can enter the total deal amount.
- DO: make label and description text concise and clear.
- DO: include placeholder text to help users understand what's expected in the field.
- DO: indicate if there is a minimum or maximum number requirement.
- DO: indicate if a field is required.
- DO: include clear validation error messages so that users know how to fix errors.
- DON'T: use this component for long responses, such as open-ended comments or feedback. Instead, use the TextArea component.
- DON'T: use placeholder text for critical information, as it will disappear once users begin to type. Critical information should be placed in the label and descriptive text, with additional context in the tooltip if needed.
Thank you for your feedback, it means a lot to us.
This form is used for documentation feedback only. Learn how to get help with HubSpot.