3 Ways to Use Custom Coded Workflow Actions With Operations Hub
On April 21st, 2021, HubSpot introduced the newest addition to the family, Operations Hub.
Designed as a full toolkit for operations professionals, this new Hub supercharges HubSpot’s CRM and makes it easier to connect, clean, and automate your customer data in one connected platform. The result: a more efficient, aligned, and agile business, an unhindered and strategic ops team, and a friction-free customer experience.
With Operations Hub, you can enjoy:
- A truly connected CRM: Keep your customer data in sync across all your business apps, two ways and in real-time — no third-party integration tools required. Save hours of manual data entry for your ops teams, and empower and align your teams with dependable, consistent, and up-to-date data.
- A database that cleans itself: Your customer experience is only as clean as the data that powers it. With Operations Hub, ensuring data quality isn’t just easy; it’s automatic. Declutter date properties, clean up country codes, polish up phone number fields, and more.
- Automation as flexible as your imagination: As online touchpoints multiply, automation keeps your team efficient and your customer experience consistent. With Operations Hub, set up custom automation to remove friction from your entire business process, adapt to ever-changing customer needs, and ensure your company runs smoothly as it scales.
How Will This Post Help You as a Developer?
In this post, we're going to dig deeper into the flexible automation that is made possible by a new feature called custom coded workflow actions. With this feature, you can write JavaScript code in a NodeJS 12.x runtime environment to perform various processes that streamline your organization's operations. We'll look at specific examples of how to:
- Validate an email address using an email validation service
- Enrich company data using a data enrichment service
- Manage a customer referral program with HubSpot
However, before we dive into these examples, let's start by breaking down how custom coded workflow actions work. (Skip straight to the examples section here).
Anatomy of a Custom Workflow Action
When you add a new custom coded workflow action to your workflow in HubSpot you'll be provided with a template to get up and running that looks like this:
There are a few important parts to be mindful of in this template:
- exports.main() - This is a function that is called when a custom coded action is executed. Regardless of what you are trying to do, this is where all of your JavaScript will go.
- event object - You'll notice that we pass an argument "event" to the exports.main() function. This object contains various pieces of information for the custom coded action to leverage, for instance the type of object enrolled in the workflow and the ID of this object. If we want, we can use this to pull additional information with the CRM API.
- callback() - This function is used to pass data back to the workflow after the workflow action has finished executing. The information stored within the callback is mapped to "data outputs" which can be used at later stages in the workflow.
Additional Libraries
There are a number of NodeJS libraries that can be used to add more functionality to your custom coded actions (full list can be found here). These libraries are loaded by using a require() function at the top of your code. The most popular library is the "request" library which allows you to make HTTP requests to other services. In the screenshot above you'll notice we are loading the HubSpot API client library in order to leverage the CRM APIs within the custom coded action.
Managing Secrets
Another important point is that you can also add your own secrets (API keys) to be used by your custom coded actions. This is done by clicking on "Manage secrets":
The advantage of using this functionality is that you can create secrets which can be referenced within your actions without having to disclose the actual information, which could present a security risk. You're able to reference the secrets as environment variables using: process.env.SECRET_NAME.
Data Outputs
When you create a custom coded workflow action, you can define a number of data outputs that can be used as an input when using the "Copy property action" later in the workflow. This is helpful if you want to copy information from a third party into a custom property within HubSpot.
When creating these outputs you can set the data type (i.e whether it's a String, Number, Enumeration, Boolean, Datetime, Date, or Phone Number).
PRO TIP: If you are dealing with a large amount of data you may consider using the CRM APIs directly within your custom coded action and avoid having to use the "Copy property action" at all. However bear in mind if you do this you'll need to be mindful of HubSpot’s API limits. In the examples further down I've opted to use the CRM APIs as I am dealing with a large number of fields.
Custom Coded Workflow Action Examples
Now that we've explored the inner workings of a custom coded workflow action, let's take a look at some practical use cases. Below you'll find walkthrough videos, sample code, and a few other details for each example. Please bear in mind that this is by no means an extensive list of what is possible. I just want to give a taste to help ignite your creativity.
Example 1: Validating an Email Address Using an Email Validation Service
Imagine a contact is created in HubSpot by a user or when someone fills in a form. Wouldn't it be fantastic if you could validate the email address for the contact before sending out an email marketing campaign? With Operations Hub and custom coded workflow actions you can. Here is a video of how to do it:

Here is the sample code from the video:
In this example, I decided to use Kickbox for email validation, specifically their "email verification API." However, there are many other services out there that do the same thing, such as ZeroBounce, MailerCheck, and Debounce.
The benefit of implementing a solution like this is that it helps to keep your IP reputation and Sender reputation high, which has a positive impact on email deliverability. It also provides additional insights into emails entered into the CRM, ensuring you're making the most efficient use of your time from a marketing standpoint.
In the screenshot below, you can see that the information is being populated into contact properties within HubSpot, and can be used for segmentation as needed.
There are other ways on which to build upon this solution. For instance, you might decide to:
- Create an active list of contacts that populates based on the "sendex score" and use this for suppressions in future campaigns.
- Delete contacts en-masse when associated emails have a "low deliverability" rating.
- Trigger a notification to contact owners informing them that there is an issue with emails associated with their customers.
Example 2: Enriching Company Data Using a Data Enrichment Service
Are you currently using a data enrichment platform like Clearbit or ZoomInfo? If not, you should really consider doing so, as this is a great way of supplementing the data you feed into the CRM. For example, adding data on the companies your contacts work for can help you focus on target accounts and tailor your outreach for account based marketing. Here's a demo of how to do this:
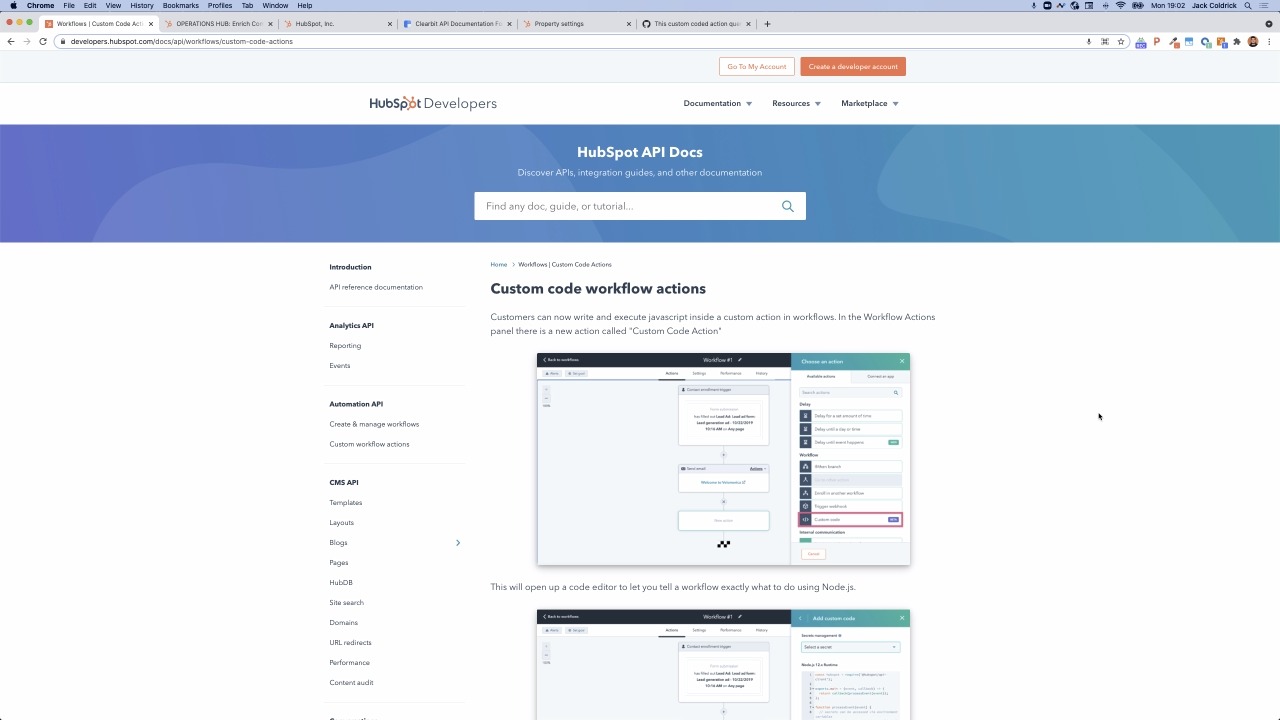
Here is the sample code from the video:
Operations Hub can be used to integrate with data enrichment systems and I chose to create this example using Clearbit, specifically their enrichment API. When a company is created this workflow queries the Clearbit API using the company domain and populates the relevant HubSpot fields.
You'll see the information I'm pulling in and storing at a company level in the image below - keep in mind you can only see some of the fields in the screenshot, but there are a lot more being mapped. For a full list of information returned from Clearbit check out the API documentation here.
Among other things, I can then use this information to:
- Create lists of companies based on various pieces of firmographic information for ad retargeting.
- Create a list of contacts who work for specific companies and reach out to them
- Use the information in these properties to create reports that add context to the data within my CRM.
Example 3) Manage a Customer Referral Program with HubSpot
One question I get asked quite regularly is, “How can I run a referral program with HubSpot?”. A few months back I recorded a video to show how you might set this up. But now with Operations Hub this is even more powerful! Take a look at this demo video:
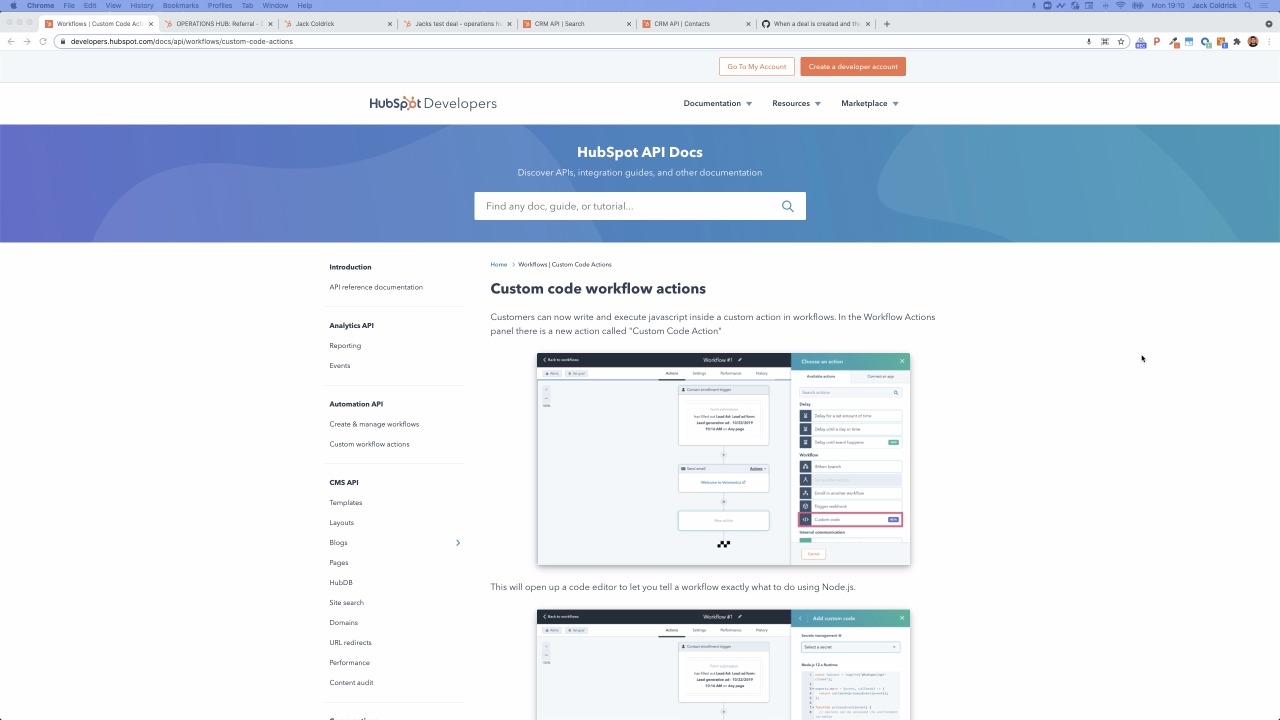
Here is the sample code from the video:
You can use a custom coded action to check the "referrer ID" of a deal against a contact in the CRM, and if a match is found, update the "total number of referrals" and the "most recent referral date" on the contact record.
You are free to leverage this information to segment contacts who have referred more than others for a rewards program. Alternatively you could display the total number of referrals on a dashboard.
That's a Wrap
I hope this article helped you understand what custom coded workflow actions are, and just how powerful they can be for automating business processes. Creativity is your only limitation when it comes to Operations Hub. For more information visit this product page.